Connect
instruct a connectable Observable to begin emitting items to its subscribers
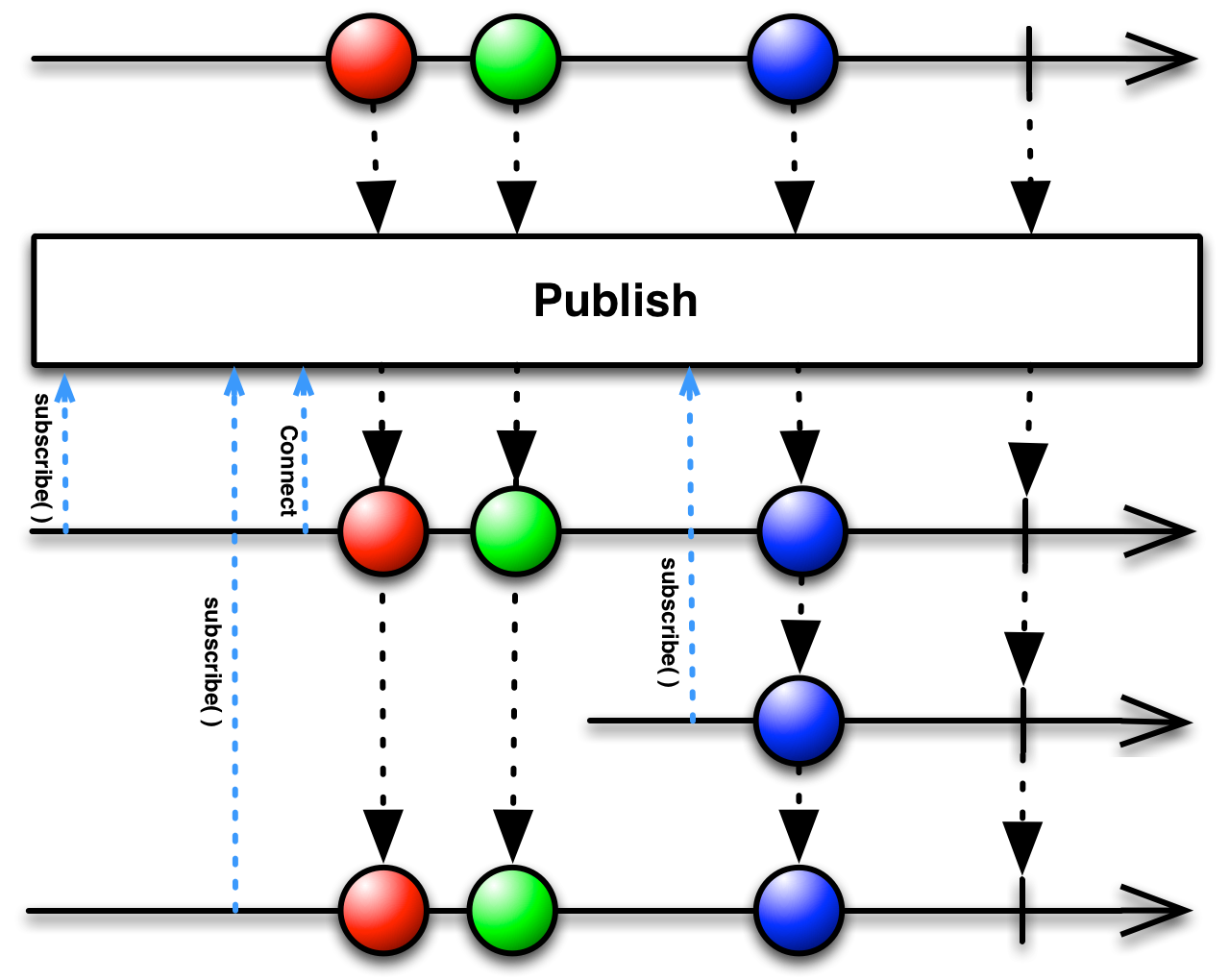
A connectable Observable resembles an ordinary Observable, except that it does not begin emitting items when it is subscribed to, but only when the Connect operator is applied to it. In this way you can wait for all intended observers to subscribe to the Observable before the Observable begins emitting items.
See Also
Language-Specific Information
RxGroovy connect
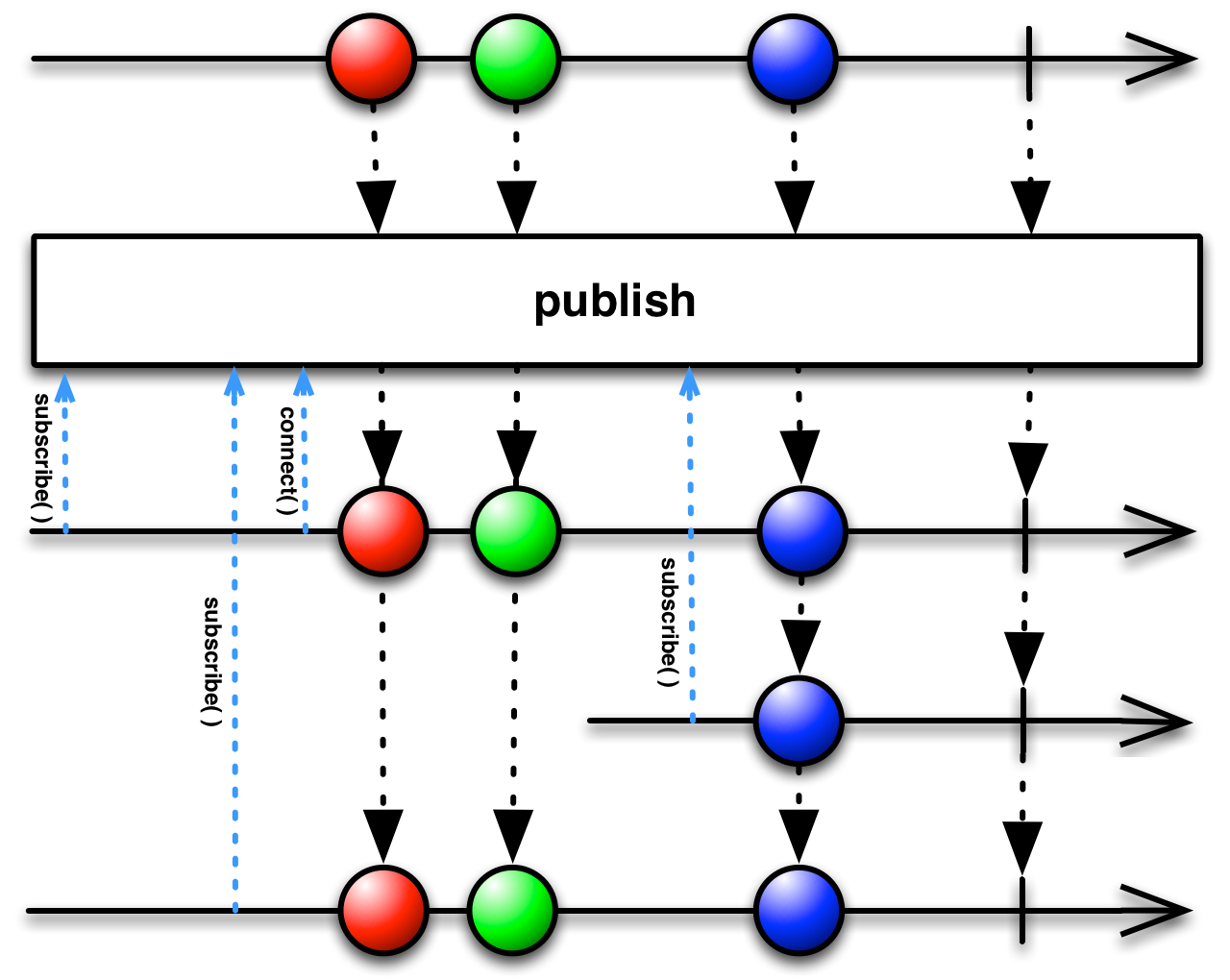
In RxGroovy, the connect
operator is a method of the ConnectableObservable
interface. You can use the publish
operator to convert an ordinary Observable into a ConnectableObservable
.
Call a ConnectableObservable
’s connect
method to instruct it to begin emitting the items from its underlying Observable to its Subscribers.
The connect
method returns a Subscription
. You can call that Subscription
object’s unsubscribe
method to instruct the Observable to stop emitting items to its Subscribers.
You can also use the connect
method to instruct an Observable to begin emitting items (or, to begin generating items that would be emitted) even before any Subscriber has subscribed to it. In this way you can turn a cold Observable into a hot one.
- Javadoc:
connect()
- Javadoc:
connect(Action1)
RxJava 1․x connect
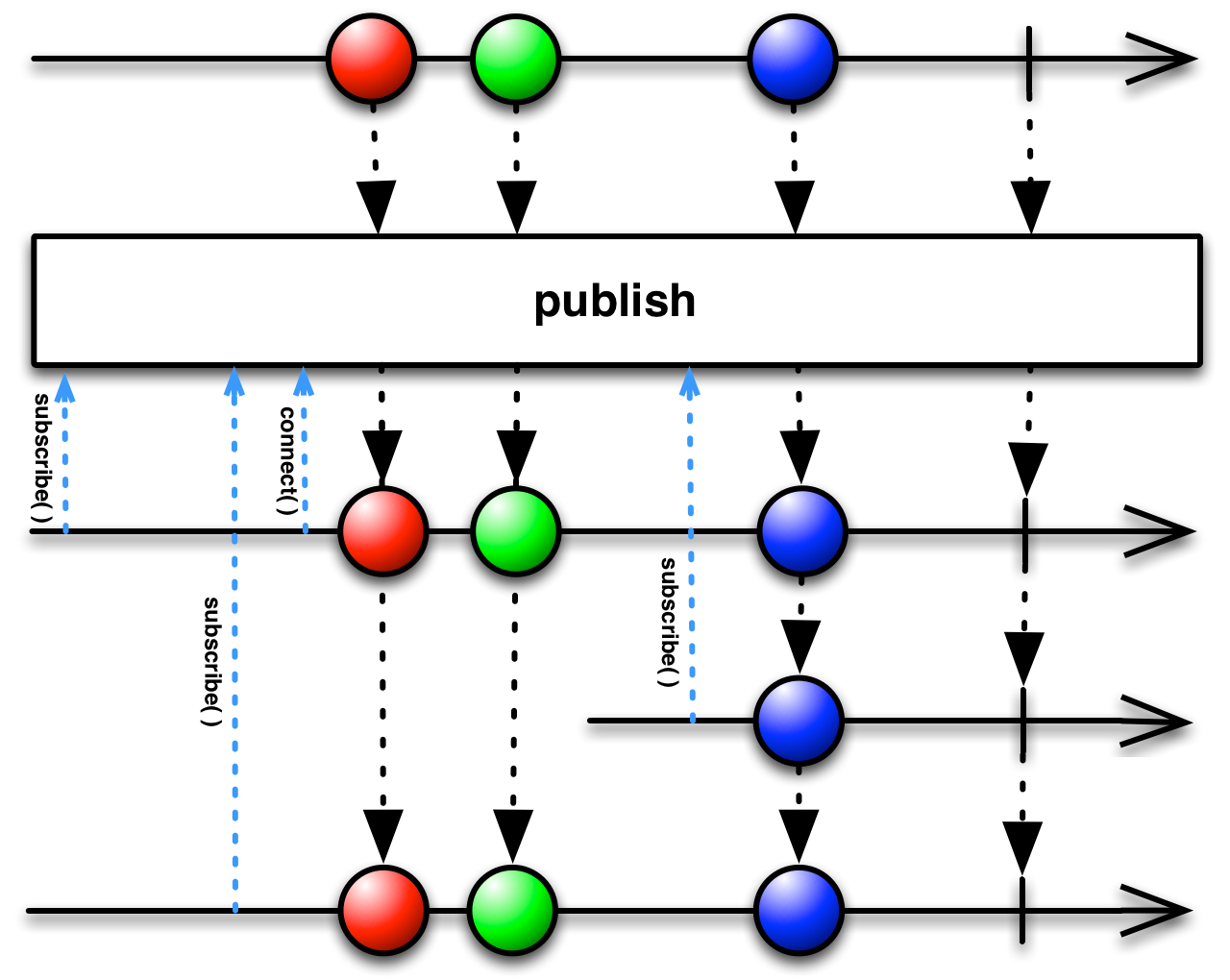
In RxJava, the connect
operator is a method of the ConnectableObservable
interface. You can use the publish
operator to convert an ordinary Observable into a ConnectableObservable
.
Call a ConnectableObservable
’s connect
method to instruct it to begin emitting the items from its underlying Observable to its Subscribers.
The connect
method returns a Subscription
. You can call that Subscription
object’s unsubscribe
method to instruct the Observable to stop emitting items to its Subscribers.
You can also use the connect
method to instruct an Observable to begin emitting items (or, to begin generating items that would be emitted) even before any Subscriber has subscribed to it. In this way you can turn a cold Observable into a hot one.
- Javadoc:
connect()
- Javadoc:
connect(Action1)
RxJS connect
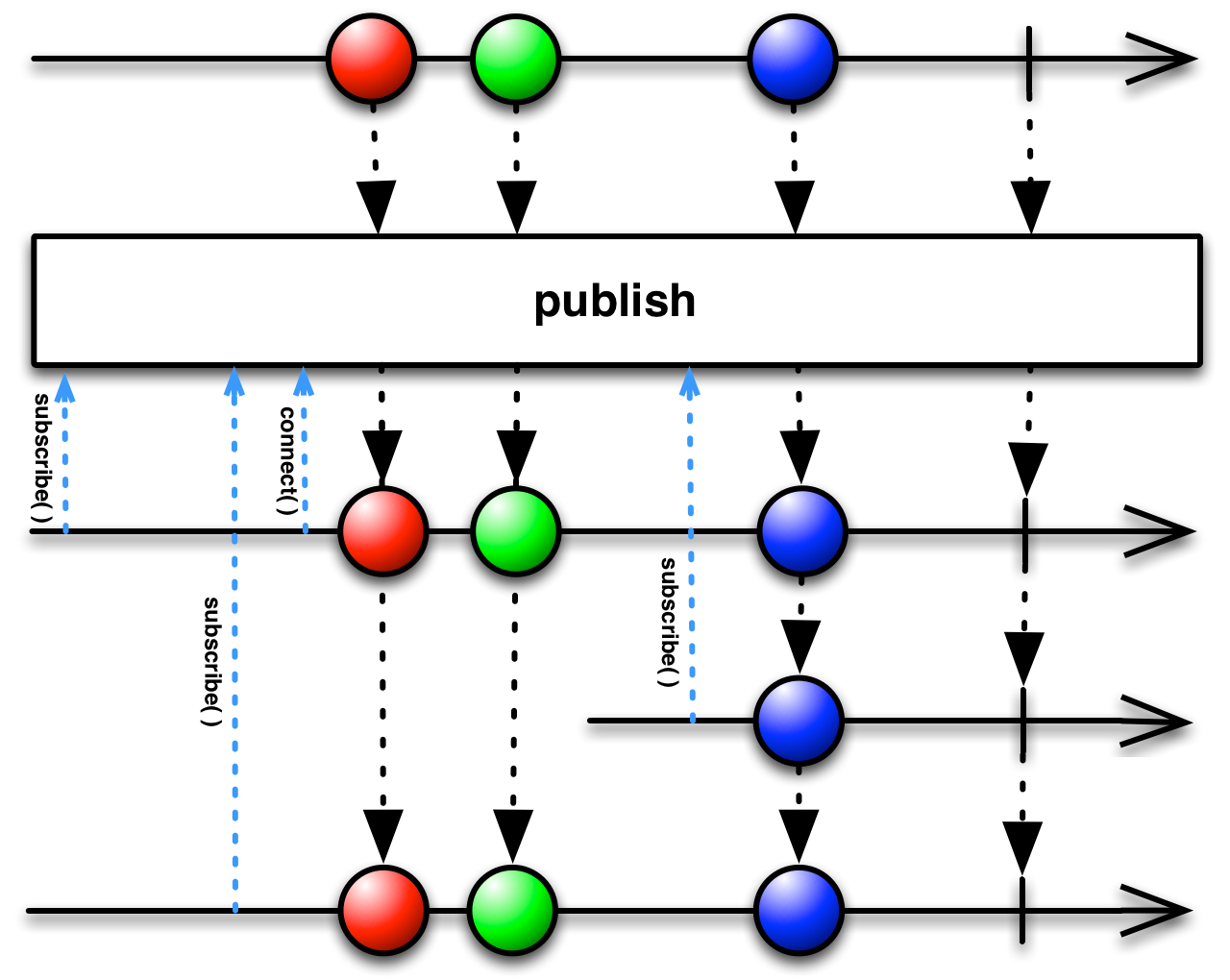
In RxJS, the connect
operator is a method of the ConnectableObservable
prototype. You can use the publish
operator to convert an ordinary Observable into a ConnectableObservable
.
Call a ConnectableObservable
’s connect
method to instruct it to begin emitting the items from its underlying Observable to its Subscribers.
The connect
method returns a Disposable
. You can call that Disposable
object’s dispose
method to instruct the Observable to stop emitting items to its Subscribers.
You can also use the connect
method to instruct an Observable to begin emitting items (or, to begin generating items that would be emitted) even before any Subscriber has subscribed to it. In this way you can turn a cold Observable into a hot one.
Sample Code
var interval = Rx.Observable.interval(1000); var source = interval .take(2) .do(function (x) { console.log('Side effect'); }); var published = source.publish(); published.subscribe(createObserver('SourceA')); published.subscribe(createObserver('SourceB')); // Connect the source var connection = published.connect(); function createObserver(tag) { return Rx.Observer.create( function (x) { console.log('Next: ' + tag + x); }, function (err) { console.log('Error: ' + err); }, function () { console.log('Completed'); }); }
Side effect Next: SourceA0 Next: SourceB0 Side effect Next: SourceA1 Next: SourceB1 Completed Completed
connect
is found in the following packages:
rx.all.js
rx.all.compat.js
rx.all.binding.js
connect
requires one of the following packages:
rx.js
rx.compat.js
rx.lite.js
rx.lite.compat.js
© ReactiveX contributors
Licensed under the Apache License 2.0.
http://reactivex.io/documentation/operators/connect.html