Empty
create an Observable that emits no items but terminates normally
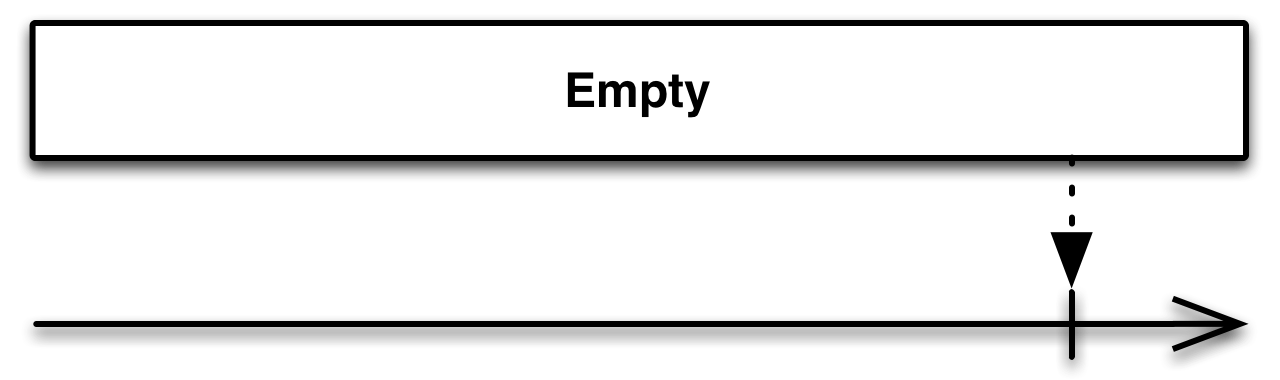
Never
create an Observable that emits no items and does not terminate
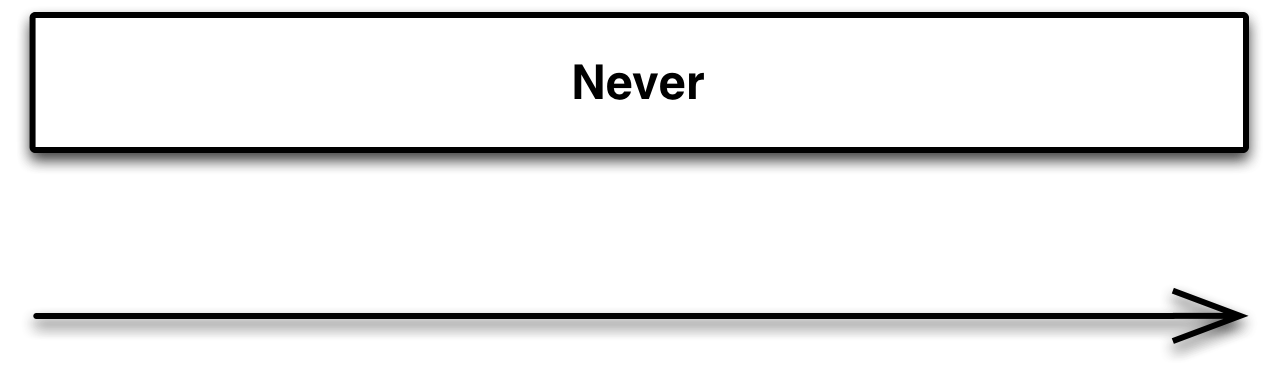
Throw
create an Observable that emits no items and terminates with an error
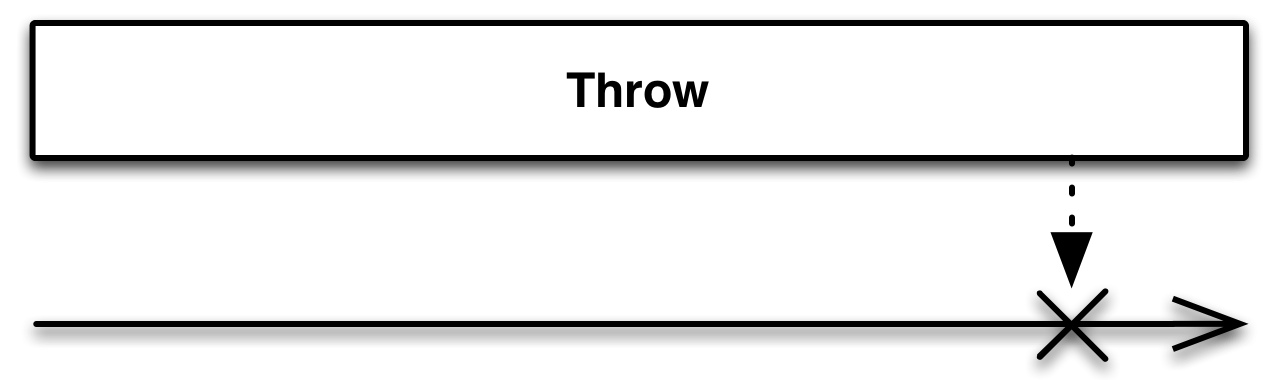
The Empty, Never, and Throw operators generate Observables with very specific and limited behavior. These are useful for testing purposes, and sometimes also for combining with other Observables or as parameters to operators that expect other Observables as parameters.
See Also
Language-Specific Information
RxGroovy empty never error
RxGroovy implements these operators as empty
, never
, and error
. The error
operator takes as a parameter the Throwable
with which you want the Observable to terminate.
These operators do not operate by default on any particular Scheduler, but empty
and error
optionally take a Scheduler as a parameter, and if you pass them a Scheduler they will issue their termination notifications on that Scheduler.
Sample Code
println("*** empty() ***"); Observable.empty().subscribe( { println("empty: " + it); }, // onNext { println("empty: error - " + it.getMessage()); }, // onError { println("empty: Sequence complete"); } // onCompleted ); println("*** error() ***"); Observable.error(new Throwable("badness")).subscribe( { println("error: " + it); }, // onNext { println("error: error - " + it.getMessage()); }, // onError { println("error: Sequence complete"); } // onCompleted ); println("*** never() ***"); Observable.never().subscribe( { println("never: " + it); }, // onNext { println("never: error - " + it.getMessage()); }, // onError { println("never: Sequence complete"); } // onCompleted ); println("*** END ***");
*** empty() *** empty: Sequence complete *** error() *** error: error - badness *** never() *** *** END ***
- Javadoc:
empty()
- Javadoc:
never()
- Javadoc:
error(throwable)
RxJava 1․x empty never error
RxJava 1.x implements these operators as empty
, never
, and error
. The error
operator takes as a parameter the Throwable
with which you want the Observable to terminate.
These operators do not operate by default on any particular Scheduler, but empty
and error
optionally take a Scheduler as a parameter, and if you pass them a Scheduler they will issue their termination notifications on that Scheduler.
- Javadoc:
empty()
- Javadoc:
never()
- Javadoc:
error(throwable)
RxJava 2․x empty never error
RxJava 2.x implements these operators as empty
, never
, and error
. The error
operator takes as a parameter the Throwable
with which you want the Observable to terminate, or a Callable
that returns such a Throwable
.
These operators do not operate by default on any particular Scheduler.
- Javadoc:
empty()
- Javadoc:
never()
- Javadoc:
error(Callable)
- Javadoc:
error(Throwable)
RxJS empty never throw
RxJS implements these operators as empty
, never
, and throw
.
Sample Code
var source = Rx.Observable.empty(); var subscription = source.subscribe( function (x) { console.log('Next: ' + x); }, function (err) { console.log('Error: ' + err); }, function () { console.log('Completed'); });
Completed
// This will never produce a value, hence never calling any of the callbacks var source = Rx.Observable.never(); var subscription = source.subscribe( function (x) { console.log('Next: ' + x); }, function (err) { console.log('Error: ' + err); }, function () { console.log('Completed'); });
var source = Rx.Observable.return(42) .selectMany(Rx.Observable.throw(new Error('error!'))); var subscription = source.subscribe( function (x) { console.log('Next: ' + x); }, function (err) { console.log('Error: ' + err); }, function () { console.log('Completed'); });
Error: Error: error!
empty
is found in the following distributions:
rx.js
rx.all.js
rx.all.compat.js
rx.compat.js
rx.lite.js
rx.lite.compat.js
never
is found in the following distributions:
rx.js
rx.compat.js
rx.lite.js
rx.lite.compat.js
throw
is found in the following distributions:
rx.js
rx.all.js
rx.compat.js
rx.lite.js
rx.lite.compat.js
RxPHP empty never error
RxPHP implements this operator as empty
.
Returns an empty observable sequence.
Sample Code
//from https://github.com/ReactiveX/RxPHP/blob/master/demo/empty/empty.php $observable = \Rx\Observable::empty(); $observable->subscribe($stdoutObserver);
Complete!
RxPHP also has an operator never
.
Returns a non-terminating observable sequence, which can be used to denote an infinite duration.
Sample Code
//from https://github.com/ReactiveX/RxPHP/blob/master/demo/never/never.php $observable = \Rx\Observable::never(); $observable->subscribe($stdoutObserver);
RxPHP also has an operator error
.
Returns an observable sequence that terminates with an exception.
Sample Code
//from https://github.com/ReactiveX/RxPHP/blob/master/demo/error-observable/error-observable.php $observable = Rx\Observable::error(new Exception('Oops!')); $observable->subscribe($stdoutObserver);
Exception: Oops!
© ReactiveX contributors
Licensed under the Apache License 2.0.
http://reactivex.io/documentation/operators/empty-never-throw.html