ObserveOn
specify the Scheduler on which an observer will observe this Observable
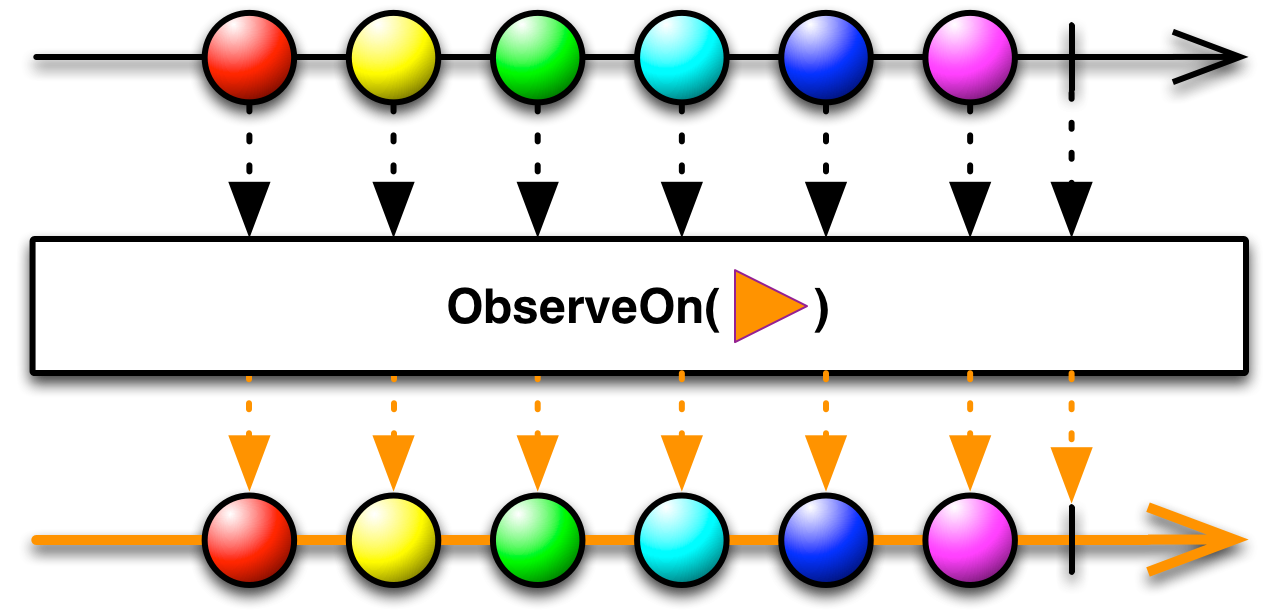
Many implementations of ReactiveX use “Scheduler
s” to govern an Observable’s transitions between threads in a multi-threaded environment. You can instruct an Observable to send its notifications to observers on a particular Scheduler by means of the ObserveOn operator.
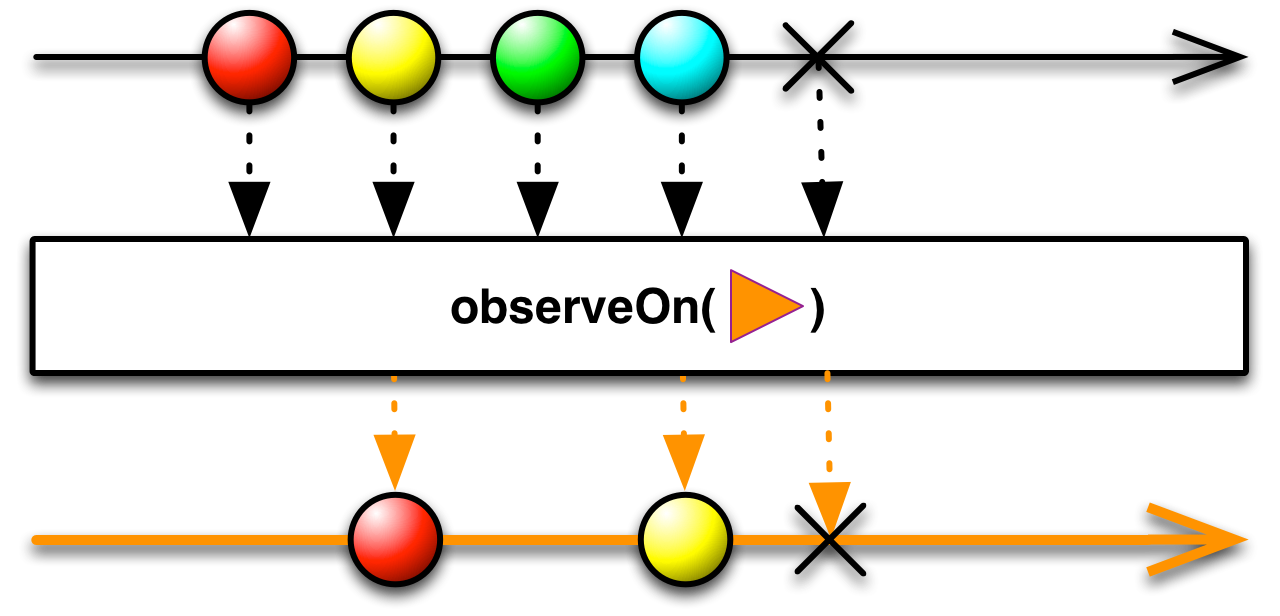
Note that ObserveOn will forward an onError
termination notification immediately if it receives one, and will not wait for a slow-consuming observer to receive any not-yet-emitted items that it is aware of first. This may mean that the onError
notification jumps ahead of (and swallows) items emitted by the source Observable, as in the diagram above.
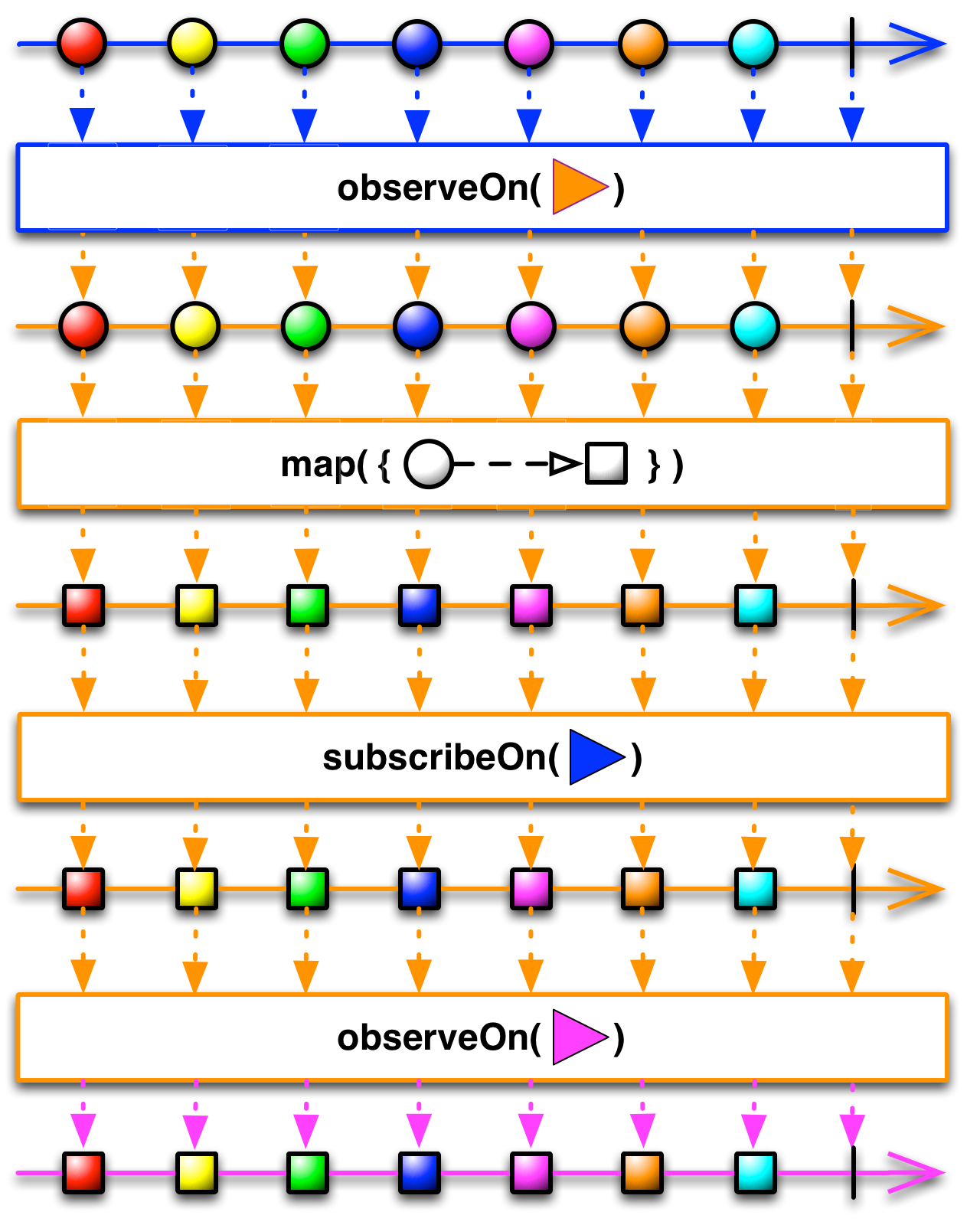
The SubscribeOn operator is similar, but it instructs the Observable to itself operate on the specified Scheduler, as well as notifying its observers on that Scheduler.
By default, an Observable and the chain of operators that you apply to it will do its work, and will notify its observers, on the same thread on which its Subscribe
method is called. The SubscribeOn operator changes this behavior by specifying a different Scheduler on which the Observable should operate. The ObserveOn operator specifies a different Scheduler that the Observable will use to send notifications to its observers.
As shown in this illustration, the SubscribeOn operator designates which thread the Observable will begin operating on, no matter at what point in the chain of operators that operator is called. ObserveOn, on the other hand, affects the thread that the Observable will use below where that operator appears. For this reason, you may call ObserveOn multiple times at various points during the chain of Observable operators in order to change on which threads certain of those operators operate.
See Also
Scheduler
- SubscribeOn
- Rx Workshop: Schedulers
- RxJava Threading Examples by Graham Lea
- Introduction to Rx: SubscribeOn and ObserveOn
- RxJava: Understanding observeOn() and subscribeOn() by Thomas Nield
- Advanced Reactive Java: SubscribeOn and ObserveOn by Dávid Karnok
Language-Specific Information
RxGroovy observeOn
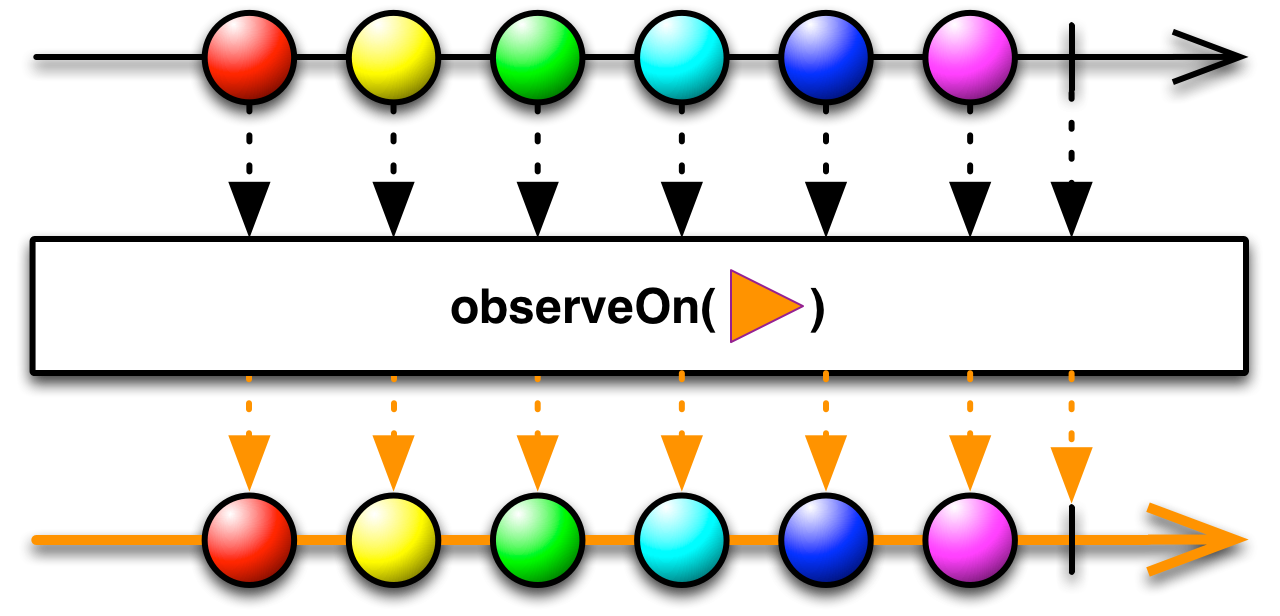
To specify on which Scheduler the Observable should invoke its observers’ onNext
, onCompleted
, and onError
methods, use the observeOn
operator, passing it the appropriate Scheduler
.
- Javadoc:
observeOn(Scheduler)
RxJava 1․x observeOn
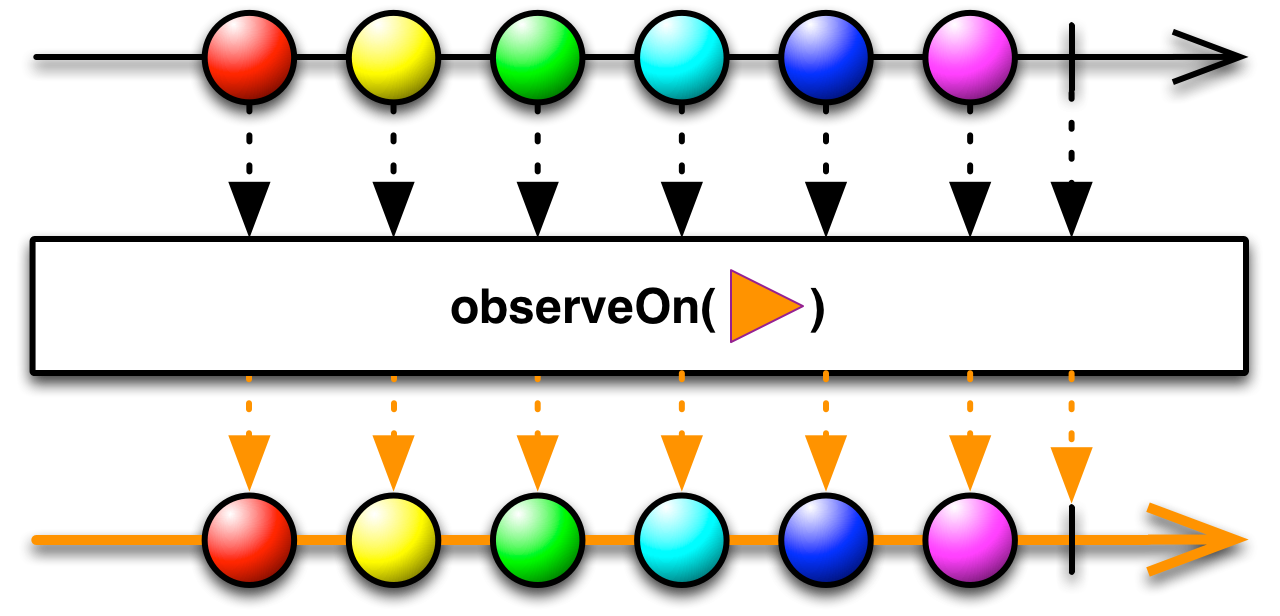
To specify on which Scheduler the Observable should invoke its observers’ onNext
, onCompleted
, and onError
methods, use the observeOn
operator, passing it the appropriate Scheduler
.
- Javadoc:
observeOn(Scheduler)
RxJS observeOn
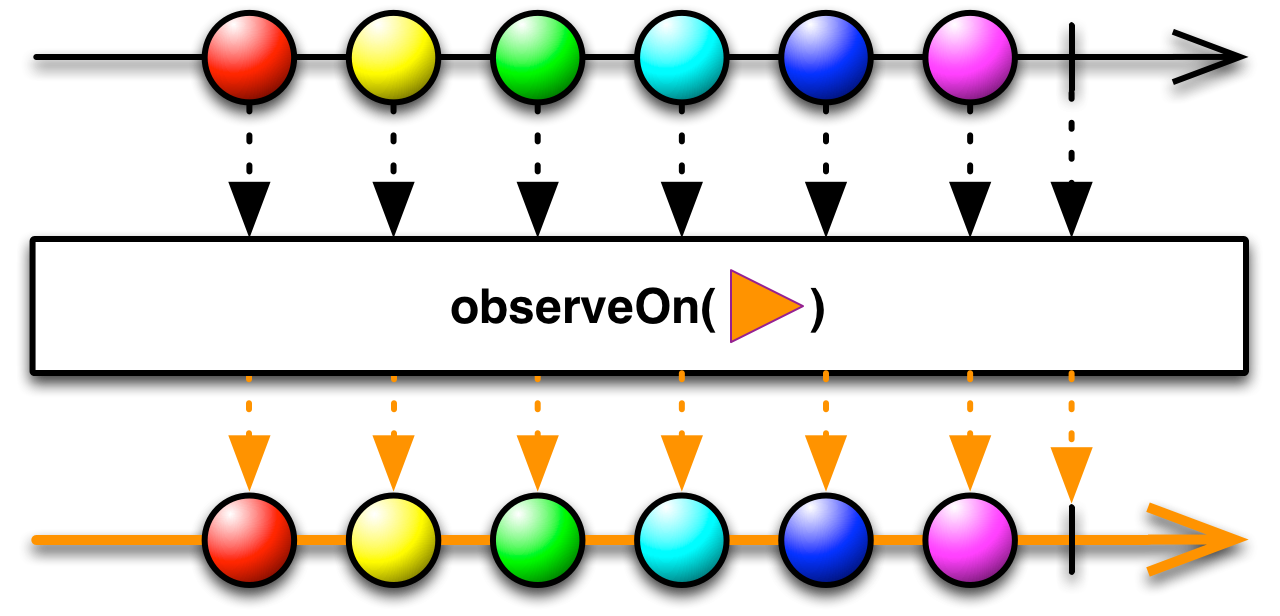
To specify on which Scheduler the Observable should invoke its observers’ onNext
, onCompleted
, and onError
methods, use the observeOn
operator, passing it the appropriate Scheduler
.
Sample Code
/* Change from immediate scheduler to timeout */ var source = Rx.Observable.return(42, Rx.Scheduler.immediate) .observeOn(Rx.Scheduler.timeout); var subscription = source.subscribe( function (x) { console.log('Next: ' + x); }, function (err) { console.log('Error: ' + err); }, function () { console.log('Completed'); });
Next: 42 Completed
observeOn
is found in each of the following distributions:
rx.js
rx.all.js
rx.all.compat.js
rx.compat.js
rx.lite.js
rx.lite.compat.js
© ReactiveX contributors
Licensed under the Apache License 2.0.
http://reactivex.io/documentation/operators/observeon.html